The first thing you should do is have your environment configured and have the web extensions for MOSS. Please see the article located here: blogspot article.
Open Visual Studio and create a new project of type "web part"
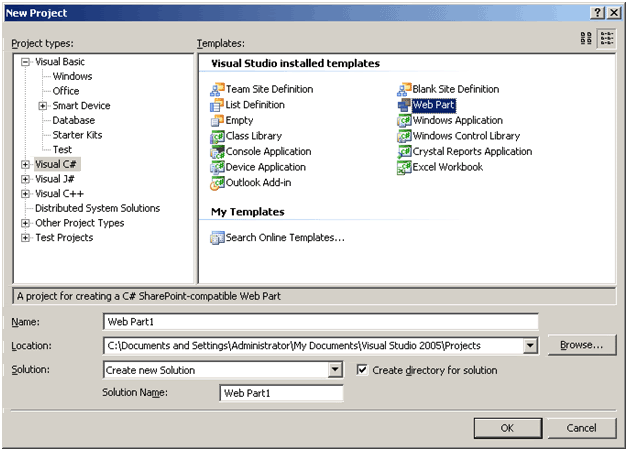
I then am going to create a sharepoint picture library and upload some pictures into it. Now, because I am going to create a rotating banner, and I am concerned about real estate, I will make sure that all the pictures are the same size. In this case I took some button images.
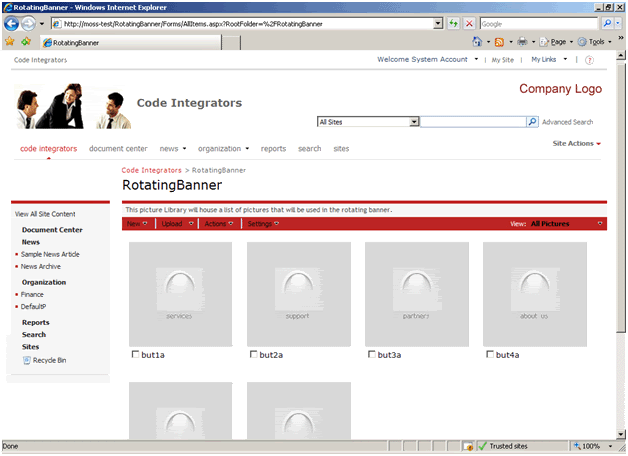
The goal at the end will be to have a random image from this selection appear on a web part on a web page within my portal.
What we are going to do next is create the *.webpart file. This file will expose and set certain properties that will be used for the webpart. Please keep in mind that many of these properties are required.
We are going to add a file of XML type to the project and call it RotatingImage.webpart.
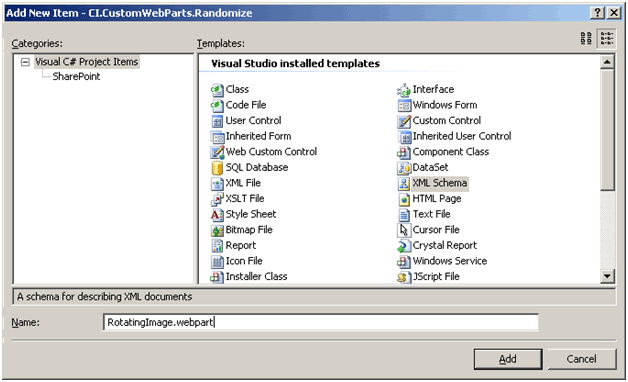
It will look something like this:
<?xml version="1.0" encoding="utf-8" ?> <property name="Description" type="string">Rotates Pictures from a Picture Library.</property> |
Obviously you would replace your public key token with the one generated from the sn command. For details on this please see my post on Code Access Security.
Next you will need to add the following to the assembly.cs file:
[assembly: AllowPartiallyTrustedCallers()] |
Before adding your web part make sure to add it to the safe controls section of the website you are going to add it to if it is not already there. If you do a deploy from visual studio and you have chosen the Sharepoint WebPart project, this should automatically be done. It would look something like this:
<SafeControl Assembly="CI.CustomWebParts.Randomize, Version=1.0.0.0, Culture=neutral, PublicKeyToken=3d0e3c375c2b1907" Namespace="CI.CustomWebParts.Randomize" TypeName="RotatingImage" Safe="True" /> |
Finally, the code. I recommend to deploy as is and add to a page first because at this point you have generated the "plumbing" of the part if you will. This will at least ensure that all is deployed correctly and you only have to worry about your custom code if it doesn't quite go as planned ;)
As I alluded to earlier in the post we are just going to pull the pictures out of a picture library and add a random one to the page. The way I did this in this example was instead of overriding the Render method that came out of the box, I deleted it and added the following code in the *.cs file:
using System; // Declarations |
Essentially what we have done here is override the child controls method and added our own image to the controls collection. You could essentially add any control you wished to this collection and it would render.
Go ahead and add your new web part to a page if you have not already done so and poof! You now have a randomized picture from your picture library. I know it probably didn't work the first time but if it did great!